11.4: Brightness Mirror - p5.js Tutorial
Education
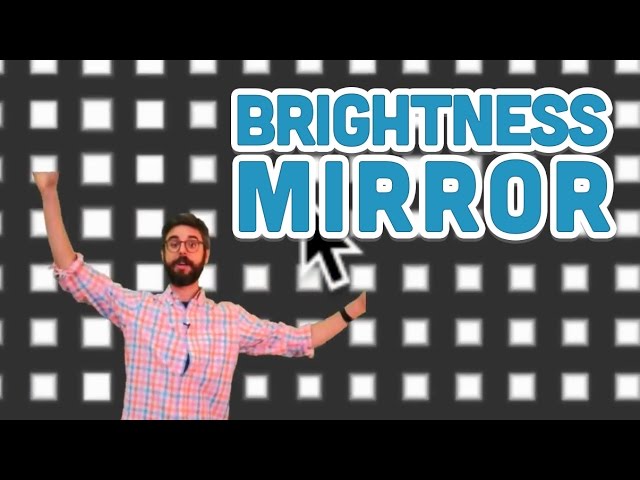
11.4: Brightness Mirror - p5.js Tutorial
In this tutorial, we will learn how to create a brightness mirror using p5.js. We will start by capturing a live video stream and then manipulate the pixel values based on their brightness to create an abstract mirror effect. The tutorial will cover concepts such as creating a video DOM element, accessing and manipulating pixel values, and rendering the manipulated pixels onto a canvas.
Step 1: Capturing the Video and Manipulating Pixels
To begin, we will create a video DOM element using the createCapture()
function in p5.js. We will set the size of the video to 320x240 pixels. Once the video is created, we need to load its pixels using the loadPixels()
function. With the video's pixels loaded, we can access the red, green, blue, and alpha values of each pixel and manipulate them.
In this tutorial, we will convert the video to grayscale by setting the red, green, and blue values of each pixel to their average. We will also set the alpha value to 255. This will create a grayscale representation of the video where brighter pixels will have higher values.
Step 2: Creating the Abstract Mirror Effect
Now that we have our grayscale video, we can create the abstract mirror effect by rendering rectangles on a canvas based on the brightness values of each pixel. We will iterate through each pixel and map its brightness value to a rectangle's size. Darker pixels will have smaller rectangles, while brighter pixels will have larger rectangles.
To achieve this effect, we need to define a variable called Vscale
that represents the scale of the video compared to the canvas. We will set it to 16, making the video 1/16th the size of the canvas. We will then iterate through each pixel of the low-resolution video and draw a rectangle on the canvas at a position scaled by the Vscale
value. The size of each rectangle will be determined by the mapped brightness value.
By using rectMode(CENTER)
, we can ensure that the rectangles grow from their centers, adding a symmetrical and aesthetically pleasing touch to the mirror effect.
Step 3: Reversing the Mirror effect and Finalizing the Code
By default, the mirror effect will appear reversed horizontally. To correct this, we need to modify the code to look up pixel values from the opposite side of the video. We can achieve this by using video.width - x + 1
to access the corresponding pixel on the other side. This will create a correctly mirrored effect.
Summary
In this tutorial, we learned how to create a brightness mirror using p5.js. We captured a video stream, manipulated its pixel values based on brightness, and rendered the manipulated pixels onto a canvas, creating an abstract mirror effect. The code also accounted for mirroring the effect horizontally to appear correctly on the screen.
Keywords: p5.js, brightness mirror, video capture, pixel manipulation
FAQ
Q: Can I use a different video source instead of the live stream?
A: Yes, you can replace the createCapture()
function with a different video source. You can load videos from files or use external URLs using the appropriate p5.js functions.
Q: Can I modify the way the pixels are rendered on the canvas? A: Absolutely! The code provided is just a starting point. You can experiment with different shapes, colors, or rendering techniques to create a unique mirror effect using the pixel values.
Q: How can I change the resolution of the mirror effect?
A: You can modify the Vscale
variable to change the resolution of the mirror effect. A smaller scale value will result in a higher-resolution mirror, while a larger scale value will reduce the resolution.