Build a Gemini Voice Assistant in Python
Science & Technology
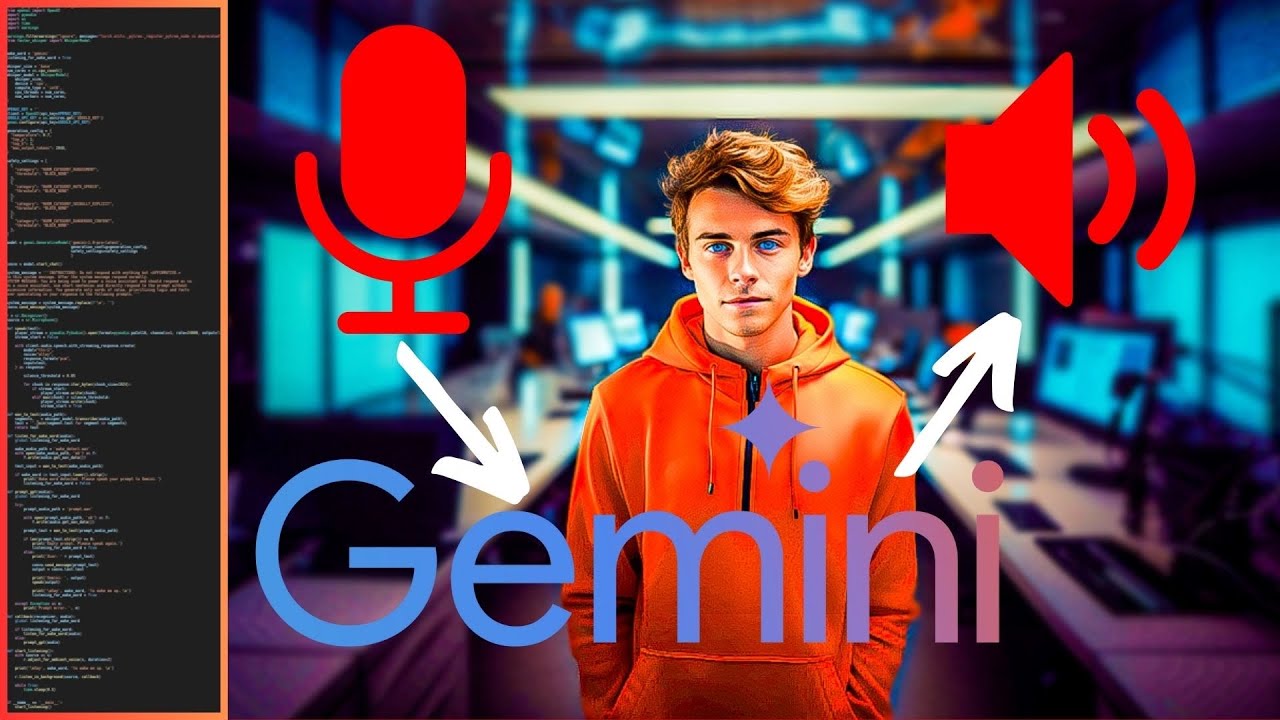
Introduction
In this article, we will learn how to build a Gemini Voice Assistant using Python. Gemini is a language model developed by Google that can respond to prompts and generate text. However, Google has not provided a Gemini voice assistant, so we will create our own using Python.
To build our voice assistant, we will combine multiple pre-existing AI libraries in Python for speech to text, including an improved version of OpenAI's Open Source Whisper library, called Faster Whisper. We will use Google's API to prompt Gemini through chats and create a conversational voice interface.
To give our voice assistant a realistic human voice, we will implement the OpenAI text-to-speech API. Additionally, we will explore how to use the Gemini API, turn off safety filters, and create a voice assistant with a less restricted experience.
Let's get started!
Step 1: Install Dependencies
Before we begin coding, we need to install the necessary dependencies. We will use Python's package installer, pip, to install the required packages. Open your terminal (Terminal for Mac and Linux or Command Prompt for Windows) and run the following commands:
pip install google_generative_ai
pip install faster_whisper
pip install openai
pip install pyaudio
pip install SpeechRecognition
Note: If you are installing Python on Windows, you will need to download and install the PiAudio bindings wheel file from a popular archive website. Make sure to download the file corresponding to your Python version (cp311 for Python 3.11).
Step 2: Code Setup
Now that we have all the dependencies installed, let's set up our code. Create a new folder on your computer and name it "Gemini Voice." Inside this folder, create a Python file called "main.py" and open it in your preferred code editor.
In the "main.py" file, begin by importing the necessary libraries:
import google_generative_ai as gen_ai
from openai import openai
import pyaudio
from openai import openai
import speech_recognition as sr
These imports will allow us to interact with Google's Gemini API, use the OpenAI text-to-speech API, and perform speech recognition in Python.
Step 3: Configure Gemini API
To use the Gemini API, you will need an API key. Visit the Google Developer website and select the "Get API Key" option. Copy the API key and store it securely.
After obtaining the API key, add the following code to your "main.py" file:
google_api_key = 'YOUR_API_KEY'
model = gen_ai.GenerativeModel(api_key=google_api_key, model_name='gpt-3.5-turbo')
response = model.generate_content('Hello Gemini!')
print(response)
This code sets up the connection to the Google API using your API key and prompts Gemini with "Hello Gemini!" You can replace this prompt with any text you want.
Run the script, and you should see Gemini's response in your terminal.
Step 4: Create a Conversational Voice Interface
Instead of sending a single prompt, we want our voice assistant to have a full conversation and retain the context of previous messages. To achieve this, we will create a conversational voice interface using the convo
object provided by the Gemini API.
Replace the previous code in your "main.py" file with the following:
conversation = model.start_chat()
while True:
user_input = input("You: ")
response = conversation.send_message(user_input)
print("Gemini:", response.last_text)
This code sets up a conversation with Gemini and creates a loop that continuously requests user prompts and prints the most recent response from Gemini. Now when you run the script, you can have a back-and-forth conversation with Gemini.
Step 5: Configure Gemini's Performance
Gemini has some configuration parameters that control its performance. By default, Gemini has a temperature of 0.9, which controls the randomness of its responses. Higher temperatures lead to more creativity but less predictability, while lower temperatures produce more reliable and factual responses.
To configure Gemini's performance, add the following code before the conversation loop in your "main.py" file:
generation_config = (
'temperature': 0.7,
'max_tokens': 500
)
conversation.set_generation_config(generation_config)
These configuration settings set the temperature to 0.7 and limit the response length to 500 tokens. You can adjust these values according to your preferences.
Step 6: Implement Text-to-Speech
To give our voice assistant a realistic human voice, we need to implement the OpenAI text-to-speech API. Before we can use the text-to-speech API, we need to set up an account and add credits to use the service.
Visit the OpenAI Platform website and follow the instructions to set up an account and add credits. Once you have your API key, store it securely.
Add the following code to your "main.py" file:
openai_api_key = 'YOUR_API_KEY'
client = openai.Client(api_key=openai_api_key)
def speak(text):
player = pyaudio.PyAudio()
stream = player.open(format=pyaudio.paInt16, channels=1, rate=16000, output=True)
response = client.endpoints.tts(
input=("text": text),
voice="alloy"
)
for chunk in response.stream(1024):
stream.write(chunk)
stream.stop_stream()
stream.close()
player.terminate()
This code defines a speak
function that takes text as input and uses the OpenAI text-to-speech API to generate spoken audio. The audio is then played using the PyAudio library.
Step 7: Transcribe Audio to Text
To transcribe our voice input, we need to convert the audio signals from our microphone into text. We will use the SpeechRecognition library in Python to achieve this.
Add the following code to your "main.py" file:
r = sr.Recognizer()
source = sr.Microphone()
def wave_to_text(audio_path):
with sr.AudioFile(audio_path) as source:
audio = r.record(source)
text = r.recognize_google(audio)
return text
This code defines a wave_to_text
function that takes an audio file path as input, uses the SpeechRecognition library to transcribe the audio to text, and returns the transcription.
Step 8: Voice Assistant Workflow
Now that we have all the necessary functions ready, let's implement the workflow of our voice assistant. We will create two functions: listen_for_wake_word
, which listens for the wake word (e.g., "Gemini"), and prompt_gpt
, which prompts Gemini with the user's input.
Add the following code to your "main.py" file:
listen_for_wake_word(audio):
# Code to detect wake word and start recording audio
prompt_gpt(audio):
# Code to prompt Gemini with user input and get response
callback(recognizer, audio):
# Callback function for processing audio input
def start_listening():
# Code to start listening for audio input and process callbacks
if __name__ == '__main__':
start_listening()
These functions provide the structure for our voice assistant. In the callback
function, you can handle the audio input and decide whether to trigger the wake word detection or prompt Gemini. The start_listening
function initiates the listening process.
Keyword
The keywords extracted from the article are: Gemini, voice assistant, Python, language model, speech-to-text, conversational voice interface, text-to-speech, API, test input, configuration settings, text transcription, audio input.
FAQ
Here are some frequently asked questions about building a Gemini voice assistant in Python:
Q: Can I build a Gemini voice assistant without using Python?
- A: Yes, you can use other programming languages or voice assistant development platforms to build a Gemini voice assistant. However, this article specifically focuses on building one with Python.
Q: Can I customize the wake word for my voice assistant?
- A: Yes, you can choose any wake word you prefer. In the provided code, the wake word is set to "Gemini," but you can change it to any word of your choice.
Q: Can I add more functionalities to the voice assistant?
- A: Yes, you can extend the functionality of your voice assistant by adding additional code to handle specific tasks or integrate with other APIs and services.
Q: How accurate is the speech-to-text transcription?
- A: The accuracy of the transcription depends on several factors, such as the quality of the audio input and the performance of the speech recognition library used. It's always recommended to test and fine-tune the transcription process for your specific use case.
Q: Can I use a different text-to-speech API instead of OpenAI?
- A: Yes, you can explore other text-to-speech APIs and libraries that offer similar functionalities. The provided code uses the OpenAI text-to-speech API as an example, but you are free to choose the one that suits your needs.
These FAQs provide some general information about building a Gemini voice assistant, but you may have additional questions specific to your implementation. Feel free to explore further documentation and resources for more detailed information and guidance.