Build an AI - Powered Voice Assistant Flutter Radio App | Android | iOS | Web
Education
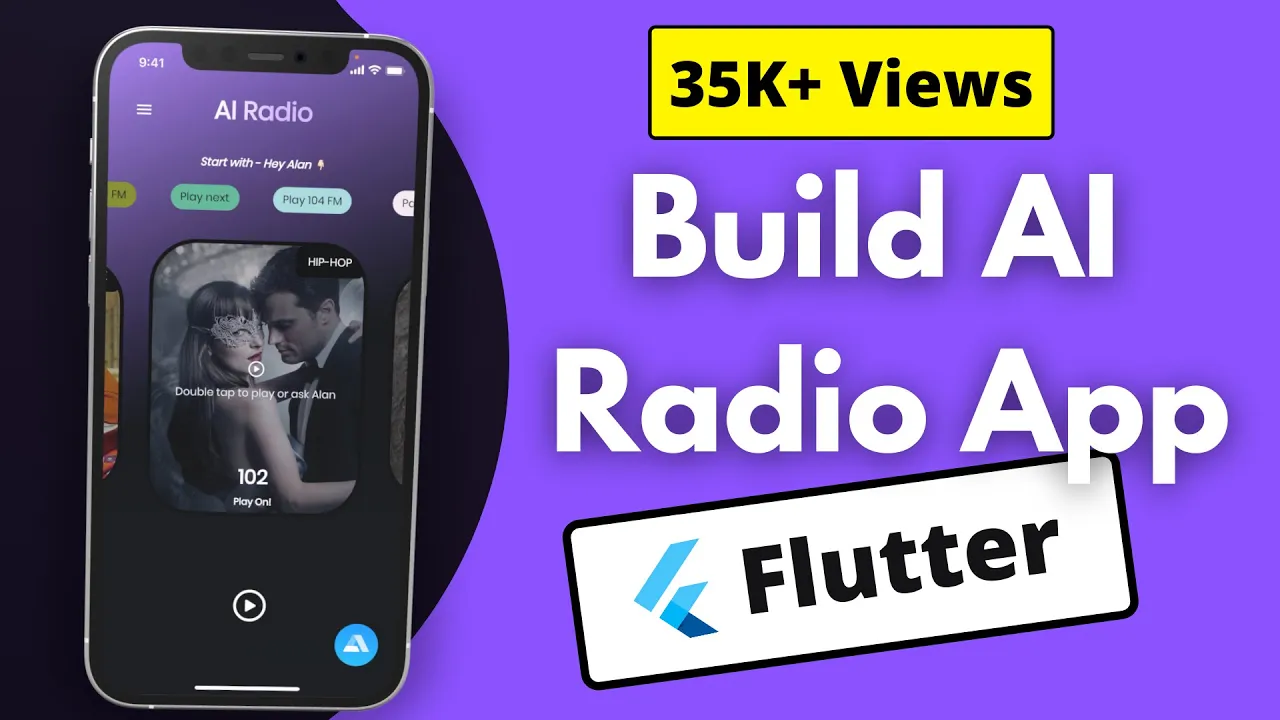
Introduction
In this comprehensive tutorial, we will learn how to create an AI-powered voice assistant radio application using Flutter. The app will allow users to play various music genres by simply asking the voice assistant named "Ellen." This guide will take you through the process of setting up the project, integrating the AI voice functionality, and building the UI from scratch.
Introduction to the AI Radio App
The AI Radio app is designed to play music based on voice commands. Users can simply say phrases such as "Hey Ellen, play some classic music," and the app will respond accordingly. This app is powered by the Flutter framework and utilizes the Allen AI voice assistant technology to handle voice commands.
Setting Up the Flutter Project
Initialize the Project: Begin by creating a new Flutter project. If you're unfamiliar with Flutter, check out beginner tutorials available online.
Install Dependencies: You will need a few dependencies to get started, including:
audio_player
: To play audio files.alan_voice
: For integrating the AI functionality.velocity_x
: For easier UI creation using Flutter.
You can add these dependencies in the pubspec.yaml
file.
- Create File Structure:
Organize your project by creating folders like
pages
,utils
, andmodels
to maintain a clean architecture.
Creating the User Interface
Build the Home Page: Create a home page with a gradient background. Use
Scaffold
andAppBar
, ensuring they offer a visually appealing design.Use Swiper for Radio Stations: Display available radio stations in a swiper format using
velocity_x
for easy navigation and visual effect.Display Music Control Options: Add play, pause, and song selection buttons. Implement a UI that tells the user which song is currently playing.
Integrating AI Voice Functionality
Setup Allen's Voice Assistant:
- Visit the Allen AI website to create a new project and obtain your unique project key.
- Integrate Allen into your Flutter application by modifying the necessary sections in your code to handle voice commands.
Define Voice Commands: Create various commands for the assistant to recognize, such as "play music," "stop," "next," "previous," and more. Use a combination of keywords and specific phrases to make the assistant's capabilities intuitive.
Command Handling in Flutter: Use Flutter's built-in capabilities alongside Allen's functionality to write code that listens for commands, processes them, and executes the corresponding actions, such as playing or stopping a song.
Adding Features to Enhance User Experience
Display Suggestions: Create a list of commands (e.g., play music, stop music, previous channel) that can be displayed to users. This can help guide them on how to use the voice commands effectively.
Drawer Menu for Radio Stations: Implement a drawer that lets users see all available channels, making navigation easier.
Responsive Design: Ensure the app is responsive and works seamlessly on both mobile and web platforms.
Testing and Debugging
Once all the features are implemented, test the app on physical devices to ensure that both the UI and the voice commands work as intended. Debug any issues related to command recognition or media playback to ensure a flawless experience.
Conclusion
By following this tutorial, you have built a fully functional AI-powered voice assistant radio application using Flutter. The integration of voice commands enhances user interaction and sets the foundation for more advanced functionalities in future applications.
Keywords
- Flutter
- AI Voice Assistant
- Radio App
- Allen
- Voice Commands
- Media Playback
- Responsive Design
FAQ
What is the purpose of the AI Radio App? The AI Radio App allows users to play music by using voice commands, offering an interactive way to enjoy radio stations.
What technologies are used in this project? The project uses Flutter for development, Allen AI for voice integration, and other packages like audio_player for media playback.
Can the app run on multiple platforms? Yes, the AI Radio App is designed to run on Android, iOS, and web platforms.
How do I set up Allen AI in my application? You'll need to create an account on the Allen AI website, generate a project key, and integrate it into your Flutter app following the tutorial.
Where can I find the source code for this tutorial? The source code will be available on GitHub for users to explore and modify as needed.