How to make advanced image recognition bots using python
Howto & Style
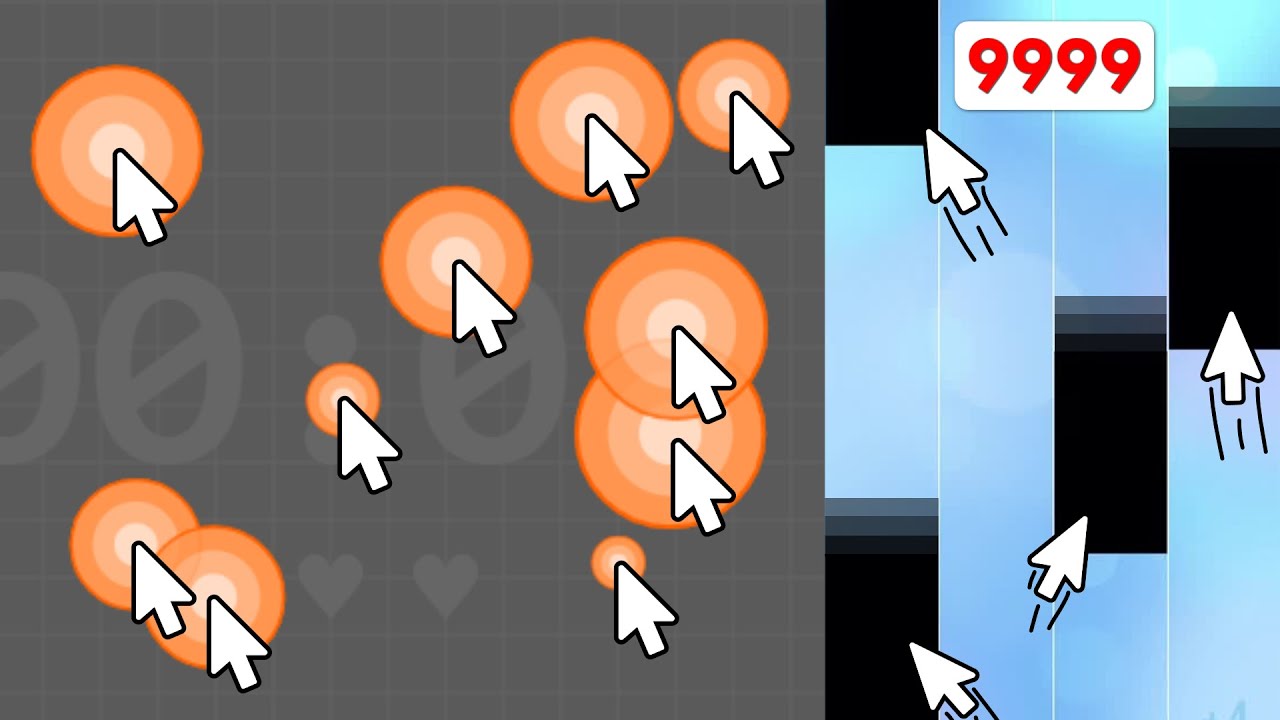
Introduction
This article will guide you through the process of creating advanced image recognition bots using Python. We'll cover two examples: a piano tile spot bot and an aim booster bot. These bots use Python libraries to automate the detection and clicking of specific elements on a screen.
Piano Tile Spot Bot
To create a piano tile spot bot, follow these steps:
- Download Python and make sure to check the "add to path" checkbox during installation.
- Open the console with administrator privileges and run the following commands to install the necessary Python libraries:
- pyautogui
- cv2
- numpy
- time
- Create a new folder and a text file named
bot.py
within that folder. - Open
bot.py
in a text editor and paste the code provided in the video description. - Adjust the pixel locations of the piano tile rows based on your screen by using the
pyautogui.displayMousePosition()
function. - Customize the click function to use the Win32 API for faster clicking.
- Implement a while loop to continuously check the pixel color of each row and click if the pixel is black.
- Run the script, switch to the piano tiles game, and start the game.
- The bot will automatically click the black tiles as they appear.
Keywords: Python, image recognition, piano tile spot bot, pyautogui, Win32 API
Aim Booster Bot
To create an aim booster bot, follow these steps:
- Go to aimbooster.com and enable Flash Player.
- Create a new file named
aimbooster.py
and paste the necessary libraries. - Follow the steps outlined in the piano tile spot bot to customize the click function and set up the while loop.
- Use the
pyautogui.screenshot()
function to take a screenshot of the game region where the circles appear. - Check the color of every fifth pixel in the screenshot to determine if it matches the color of the center of the target.
- Customize the click function to click on the target's position relative to the game region.
- Add a delay after each click to prevent misclicks.
- Run the script, switch to the aim booster game, and start the challenge.
Keywords: Python, image recognition, aim booster bot, pyautogui
FAQ
Q: Does the script work on all screen resolutions? A: Yes, the script can be adjusted to work with different screen resolutions by modifying the pixel locations and click functions accordingly.
Q: Can I customize the color detection parameters for different games? A: Absolutely! You can modify the RGB values or implement a range of values to match the specific colors used in different games.
Q: Is there a way to improve the accuracy of the bots? A: If you experience accuracy issues, you can adjust the delay after each click to ensure the bot doesn't click too quickly and potentially double-click. Tweak the delay timing until you achieve the desired accuracy.
Q: Are there other image recognition tasks I can automate using Python? A: Yes, Python offers several image recognition libraries, such as OpenCV and TensorFlow, that can be used for various automation tasks beyond simple color detection, such as object detection and facial recognition.
Q: Can I run these bots on a different operating system? A: These bots are compatible with Windows. However, with some modifications to the libraries and functions used, you can adapt them to run on other operating systems like macOS or Linux.
Keywords: FAQs, image recognition, script customization, accuracy improvement, Python, cross-platform compatibility