Image Recognition with LLaVa in Python
Science & Technology
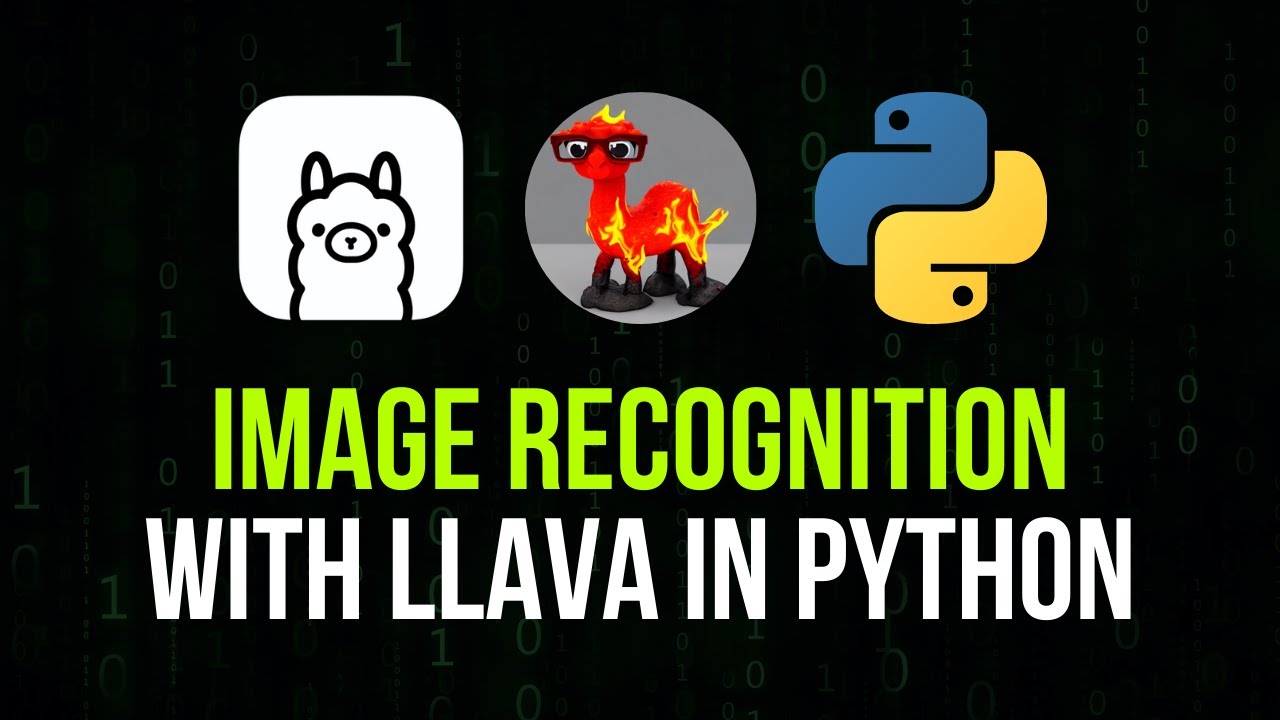
Introduction
Welcome back! In this article, we’ll explore how to use LLaVa, the Large Language and Vision Assistant, locally to perform image recognition in Python. This guide will walk you through the necessary steps to set up LLaVa on your local machine and demonstrate some basic image recognition tasks using Python.
Setting Up LLaVa
To get started with LLaVa, we first need a tool called olama. You can download it from ama.com for Mac, Linux, and Windows. For Linux users, a simple curl command will suffice.
To pull a model onto your system, open your command line interface and type:
olama pull llava:<model_size>
In this case, you can choose from various model sizes like 7 billion, 13 billion, or 34 billion parameters. For balance between performance and resource consumption, I recommend going with the 13 billion parameter model, but if your system struggles, you can use the 7 billion version instead.
For example, the command for pulling the 13 billion model would look like:
olama pull llava:13b
After downloading the model, install the olama Python package:
pip install olama
Performing Image Recognition
In this tutorial, we have prepared four copyright-free images to test LLaVa's capabilities. We’ll send these images to LLaVa and prompt it with various questions to see how well it recognizes and describes the content.
Coding Usage
Here’s a basic code structure to use LLaVa for image recognition in Python:
import olama
## Introduction
response = olama.chat(
model='llava:13b', # or 'llava:7b'
messages=[
(
'role': 'user',
'content': 'Describe this image.'
)
],
images=['./image1.jpeg']
)
## Introduction
print(response['message']['content'])
Testing with Images
We tested the model using one image that was expected to describe a field of crops. The model provided a detailed response, indicating it recognized the elements present in the image quite well.
We then changed the image to show a laptop being used by an individual, prompting it with questions about what programming language was displayed. While it initially struggled, eventually it recognized the image accurately, indicating the presence of coding.
Next, we prompted it to count the number of dogs in a separate image. Although the model inaccurately counted three dogs when there were only two, it generally performed well.
Automated Keyword Generation
An additional utility of LLaVa is to generate keywords or hashtags from an image. This can be beneficial for social media or organizing content. We prompted LLaVa to provide keywords for each image, yielding fairly accurate descriptions.
Final Observations
While the 13 billion parameter model provided more accurate results, the 7 billion model also performed admirably on various tasks. Users can adapt their choice of model depending on available system resources and required accuracy.
Conclusion
In summary, setting up LLaVa locally allows for flexible and effective image recognition in Python. By leveraging this powerful model, you can automate processes such as annotating images and generating relevant keywords.
I hope this article has been informative. If you found it helpful, please consider liking, commenting, and subscribing to the channel for future updates!
Keywords
- LLaVa
- Image Recognition
- Python
- olama
- Model Parameters
- Keyword Generation
- Image Annotation
FAQ
Q1: What is LLaVa?
A1: LLaVa stands for Large Language and Vision Assistant, a model used for image recognition tasks.
Q2: How do I install olama?
A2: You can download olama from ama.com for various operating systems, and it can be installed via command line.
Q3: Which model size should I choose?
A3: It depends on your system's capability. The 13 billion parameter model is more accurate but requires more resources. If your system struggles, the 7 billion model can be a good alternative.
Q4: Can LLaVa generate keywords from images?
A4: Yes, LLaVa can provide keywords or hashtags based on the content of images, which can be useful for social media or organization.
Q5: What kind of images did you use for testing?
A5: We used four copyright-free images featuring a field of crops, a laptop, an indoor pool, and pets (dogs and cats) to test LLaVa’s recognition capabilities.